diff --git a/Document/Message.md b/Document/Message.md
deleted file mode 100644
index c2f909c..00000000
--- a/Document/Message.md
+++ /dev/null
@@ -1,61 +0,0 @@
-## Voice Message
-```js
- const channel = client.channels.cache.get('cid');
- const attachment = new Discord.MessageAttachment(
- './test.mp3', // path file
- 'test.ogg', // must be .ogg
- {
- waveform: '=',
- duration_secs: 1, // any number you want
- },
- );
- channel.send({
- files: [attachment],
- flags: 'IS_VOICE_MESSAGE',
- });
-```
-
-## Interaction
-
-Slash Command
-
-### [Click here](https://github.com/aiko-chan-ai/discord.js-selfbot-v13/blob/main/Document/SlashCommand.md)
-
-
-
-
-## MessageEmbed ?
-- Because Discord has removed the ability to send Embeds in its API, that means MessageEmbed is unusable. But I have created a constructor that uses oEmbed with help [from this site](https://www.reddit.com/r/discordapp/comments/82p8i6/a_basic_tutorial_on_how_to_get_the_most_out_of/)
-
-
-Click to show
-
-
-Code:
-```js
-const Discord = require('discord.js-selfbot-v13');
-const w = new Discord.WebEmbed()
- .setAuthor({ name: 'hello', url: 'https://google.com' })
- .setColor('RED')
- .setDescription('description uh')
- .setProvider({ name: 'provider', url: 'https://google.com' })
- .setTitle('This is Title')
- .setURL('https://google.com')
- .setImage(
- 'https://cdn.discordapp.com/attachments/820557032016969751/959093026695835648/unknown.png',
- )
- .setVideo(
- 'https://cdn.discordapp.com/attachments/877060758092021801/957691816143097936/The_Quintessential_Quintuplets_And_Rick_Astley_Autotune_Remix.mp4',
- );
-message.channel.send({ content: `Hello world ${Discord.WebEmbed.hiddenEmbed} ${w}` });
-
-```
-### Features & Issues
-- Only works with Discord Web and Discord Client (no custom theme installed)
-- No Timestamp, Footer, Fields, Author iconURL
-- Video with Embed working
-- Can only choose between image and thumbnail
-- Description limit 350 characters
-- If you use hidden mode you must make sure your custom content is less than 1000 characters without nitro (because hidden mode uses 1000 characters + URL)
-
-
diff --git a/Document/RichPresence.md b/Document/RichPresence.md
deleted file mode 100644
index 9c29b64..00000000
--- a/Document/RichPresence.md
+++ /dev/null
@@ -1,126 +0,0 @@
-## Custom Status and RPC
-
-Custom Status
-
-```js
-const Discord = require('discord.js-selfbot-v13');
-const r = new Discord.CustomStatus()
- .setState('Discord')
- .setEmoji('π¬')
-client.user.setActivity(r);
-```
-
-
-
-Rich Presence [Custom]
-```js
-const Discord = require('discord.js-selfbot-v13');
-const r = new Discord.RichPresence()
- .setApplicationId('817229550684471297')
- .setType('STREAMING')
- .setURL('https://youtube.com/watch?v=dQw4w9WgXcQ')
- .setState('State')
- .setName('Name')
- .setDetails('Details')
- .setParty({
- max: 9,
- current: 1,
- id: Discord.getUUID(),
- })
- .setStartTimestamp(Date.now())
- .setAssetsLargeImage('929325841350000660')
- .setAssetsLargeText('Youtube')
- .setAssetsSmallImage('895316294222635008')
- .setAssetsSmallText('Bot')
- .addButton('name', 'https://link.com/')
-client.user.setActivity(r);
-```
-
-
-Rich Presence with Spotify
-```js
-const Discord = require('discord.js-selfbot-v13');
-const r = new Discord.SpotifyRPC(client)
- .setAssetsLargeImage("spotify:ab67616d00001e02768629f8bc5b39b68797d1bb") // Image ID
- .setAssetsSmallImage("spotify:ab6761610000f178049d8aeae802c96c8208f3b7") // Image ID
- .setAssetsLargeText('ζͺζ₯θΆε± (vol.1)') // Album Name
- .setState('Yunomi; Kizuna AI') // Artists
- .setDetails('γγγγγγΌγ') // Song name
- .setStartTimestamp(Date.now())
- .setEndTimestamp(Date.now() + 1_000 * (2 * 60 + 56)) // Song length = 2m56s
- .setSongId('667eE4CFfNtJloC6Lvmgrx') // Song ID
- .setAlbumId('6AAmvxoPoDbJAwbatKwMb9') // Album ID
- .setArtistIds('2j00CVYTPx6q9ANbmB2keb', '2nKGmC5Mc13ct02xAY8ccS') // Artist IDs
-client.user.setActivity(r);
-```
-
-
-
-
-You can now add custom images for RPC !
-
-> Tutorial:
-
-## Method 1: (Discord URL, v2.3.78+)
-
-```js
-const Discord = require('discord.js-selfbot-v13');
-const r = new Discord.RichPresence()
- .setApplicationId('817229550684471297')
- .setType('PLAYING')
- .setURL('https://youtube.com/watch?v=dQw4w9WgXcQ')
- .setState('State')
- .setName('Name')
- .setDetails('Details')
- .setParty({
- max: 9,
- current: 1,
- id: Discord.getUUID(),
- })
- .setStartTimestamp(Date.now())
- .setAssetsLargeImage('https://cdn.discordapp.com/attachments/820557032016969751/991172011483218010/unknown.png')
- .setAssetsLargeText('Youtube')
- .setAssetsSmallImage('895316294222635008')
- .setAssetsSmallText('Bot')
- .addButton('name', 'https://link.com/')
-client.user.setActivity(r);
-```
-
-
-
-## Method 2 (Custom URL, 2.3.78+)
-
-```js
-const Discord = require('discord.js-selfbot-v13');
-const rpc = new Discord.RichPresence();
-const imageSet = await Discord.RichPresence.getExternal(client, '820344593357996092', 'https://musedash.moe/covers/papipupipupipa_cover.hash.93ae31d41.png', 'https://musedash.moe/covers/lights_of_muse_cover.hash.1c18e1e22.png')
-rpc
- .setApplicationId('820344593357996092')
- .setType('PLAYING')
- .setState('pa pi pu pi pu pi pa - γγγγ½γ‘ With η«η§')
- .setName('Muse Dash')
- .setDetails('Hard - Lvl.8')
- .setAssetsLargeImage(imageSet[0].external_asset_path)
- .setAssetsSmallImage(imageSet[1].external_asset_path)
-client.user.setActivity(rpc);
-```
-
-
-
-How to get the Assets ID and Name of the bot (application) ?
-
-- Bot:
-```js
-const bot = await client.users.fetch('BotId');
-const asset = await bot.application.fetchAssets();
-// asset: Array
-// Document: https://discordjs-self-v13.netlify.app/#/docs/docs/main/typedef/ApplicationAsset
-```
-
-
-- Application
-> Using Browser (Chrome) with URL: `https://discord.com/api/v9/oauth2/applications/{applicationId}/assets`
-
-
-- More:
- - You can change the status 5 times / 20 seconds!
diff --git a/Document/SamsungRPC.md b/Document/SamsungRPC.md
deleted file mode 100644
index ff89045..00000000
--- a/Document/SamsungRPC.md
+++ /dev/null
@@ -1,16 +0,0 @@
-
-
-```js
-const { Client } = require('discord.js-selfbot-v13');
-
-const client = new Client();
-
-client.on('ready', async () => {
- client.user.setSamsungActivity('com.YostarJP.BlueArchive', 'START');
- // client.user.setSamsungActivity('com.miHoYo.bh3oversea', 'UPDATE');
- // client.user.setSamsungActivity('com.miHoYo.GenshinImpact', 'STOP');
-});
-
-client.login('token');
-```
-
diff --git a/Document/SlashCommand.md b/Document/SlashCommand.md
deleted file mode 100644
index b7ca1b7..00000000
--- a/Document/SlashCommand.md
+++ /dev/null
@@ -1,44 +0,0 @@
-# Slash command
-
-# Slash Command (no options)
-
-### Demo
-
-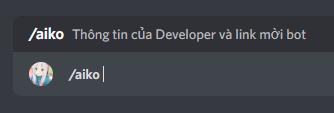
-
-### Code
-
-```js
-await message.channel.sendSlash('botid', 'aiko')
-```
-
-# Slash Command + Sub option (group)
-
-### Demo
-
-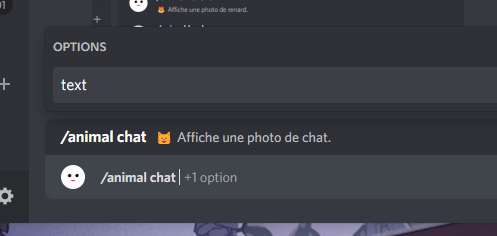
-
-### Code test
-
-```js
-await message.channel.sendSlash('450323683840491530', 'animal chat', 'bye')
-```
-
-# Slash Command with Attachment
-
-### Demo
-
-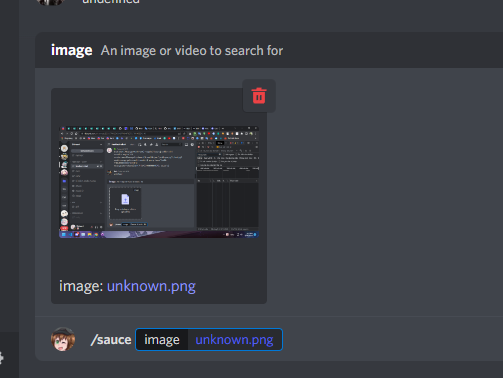
-
-### Code test
-
-```js
-const { MessageAttachment } = require('discord.js-selfbot-v13')
-const fs = require('fs')
-const a = new MessageAttachment(fs.readFileSync('./wallpaper.jpg') , 'test.jpg')
-await message.channel.sendSlash('718642000898818048', 'sauce', a)
-```
-
-### Result
-
-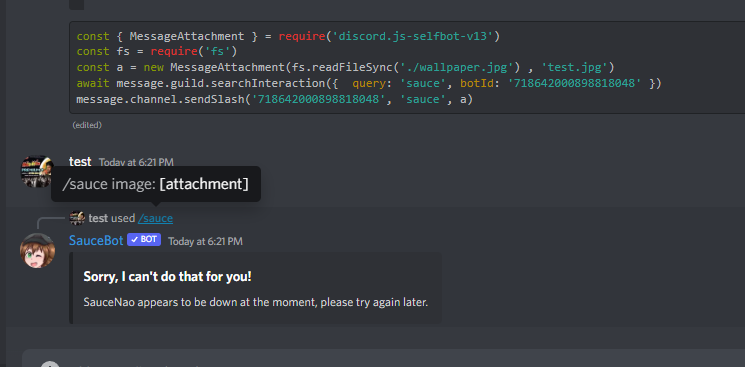
diff --git a/README.md b/README.md
index e4dbf45..57b3a06 100644
--- a/README.md
+++ b/README.md
@@ -34,25 +34,20 @@
`discord.js-selfbot-v13` is currently in maintenance mode. New features are not actively being added but existing features and new versions of discord are supported as possible. There are some major architectural changes which need to be added to improve the stability and security of the project. I don't have as much spare time as I did when I started this project, so there is not currently any plan for these improvements.
-### [Document Website (recommend)](https://discordjs-self-v13.netlify.app/)
+### [Document Website](https://discordjs-self-v13.netlify.app/)
-### [Extend Document (With Example)](https://github.com/aiko-chan-ai/discord.js-selfbot-v13/tree/main/Document)
+### [Example Code](https://github.com/aiko-chan-ai/discord.js-selfbot-v13/tree/main/examples)
## Features (User)
- [x] Message: Embeds (WebEmbed)
-- [x] User: Settings, Status, Activity, DeveloperPortal, RemoteAuth, etc.
+- [x] User: Status, Activity, RemoteAuth, etc.
- [X] Guild: Fetch Members, Join / Leave, Top emojis, ...
-- [X] Interactions: Slash Commands, Click Buttons, Menu, Modal, Context Menu, ...
+- [X] Interactions: Slash Commands, Buttons, Menu, Modal
- [X] Captcha Handler (2captcha, capmonster, custom)
- [X] Documentation
- [x] Voice & [Video stream](https://github.com/aiko-chan-ai/discord.js-selfbot-v13/issues/293)
- [ ] Everything
-### Optional packages
-
-- [2captcha](https://www.npmjs.com/package/2captcha) for solving captcha (`npm install 2captcha`)
-- [node-capmonster](https://www.npmjs.com/package/node-capmonster) for solving captcha (`npm install node-capmonster`)
-
## Installation
**Node.js 16.6.0 or newer is required**
@@ -67,11 +62,7 @@ npm install discord.js-selfbot-v13@latest
```js
const { Client } = require('discord.js-selfbot-v13');
-const client = new Client({
- // See other options here
- // https://discordjs-self-v13.netlify.app/#/docs/docs/main/typedef/ClientOptions
- // All partials are loaded automatically
-});
+const client = new Client();
client.on('ready', async () => {
console.log(`${client.user.username} is ready!`);
@@ -109,7 +100,7 @@ console.log(`%cYou now have your token in the clipboard!`, 'font-size: 16px');
## Contributing
- Before creating an issue, please ensure that it hasn't already been reported/suggested, and double-check the
-[documentation](https://discord.js.org/#/docs).
+[documentation](https://discordjs-self-v13.netlify.app/).
- See [the contribution guide](https://github.com/discordjs/discord.js/blob/main/.github/CONTRIBUTING.md) if you'd like to submit a PR.
## Need help?
diff --git a/examples/ActivityMessage.js b/examples/ActivityMessage.js
new file mode 100644
index 00000000..306f3e9
--- /dev/null
+++ b/examples/ActivityMessage.js
@@ -0,0 +1,15 @@
+const { Client } = require('../src/index');
+const client = new Client();
+
+client.on('ready', async () => {
+ console.log(`${client.user.username} is ready!`);
+ const channel = client.channels.cache.get('id');
+ channel.send({
+ activity: {
+ type: 3, // MessageActivityType.Listen
+ partyId: `spotify:${client.user.id}`,
+ },
+ });
+});
+
+client.login('token');
diff --git a/examples/Basic.js b/examples/Basic.js
new file mode 100644
index 00000000..84dbd68
--- /dev/null
+++ b/examples/Basic.js
@@ -0,0 +1,14 @@
+const { Client } = require('../src/index');
+const client = new Client();
+
+client.on('ready', async () => {
+ console.log(`${client.user.username} is ready!`);
+});
+
+client.on("messageCreate", message => {
+ if (message.content == 'ping') {
+ message.reply('pong');
+ }
+});
+
+client.login('token');
diff --git a/examples/Embed.js b/examples/Embed.js
new file mode 100644
index 00000000..29b3bfe
--- /dev/null
+++ b/examples/Embed.js
@@ -0,0 +1,41 @@
+const { Client, WebEmbed } = require('../src/index');
+const client = new Client();
+
+client.on('ready', async () => {
+ console.log(`${client.user.username} is ready!`);
+});
+
+client.on('messageCreate', message => {
+ if (message.content == 'embed_hidden_url') {
+ const embed = new WebEmbed()
+ .setAuthor({ name: 'hello', url: 'https://google.com' })
+ .setColor('RED')
+ .setDescription('description uh')
+ .setProvider({ name: 'provider', url: 'https://google.com' })
+ .setTitle('This is Title')
+ .setURL('https://google.com')
+ .setImage('https://i.ytimg.com/vi/iBP8HambzpY/maxresdefault.jpg')
+ .setRedirect('https://www.youtube.com/watch?v=iBP8HambzpY')
+ .setVideo('http://commondatastorage.googleapis.com/gtv-videos-bucket/sample/BigBuckBunny.mp4');
+ message.channel.send({
+ content: `Hello world ${WebEmbed.hiddenEmbed}${embed}`,
+ });
+ }
+ if (message.content == 'embed') {
+ const embed = new WebEmbed()
+ .setAuthor({ name: 'hello', url: 'https://google.com' })
+ .setColor('RED')
+ .setDescription('description uh')
+ .setProvider({ name: 'provider', url: 'https://google.com' })
+ .setTitle('This is Title')
+ .setURL('https://google.com')
+ .setImage('https://i.ytimg.com/vi/iBP8HambzpY/maxresdefault.jpg')
+ .setRedirect('https://www.youtube.com/watch?v=iBP8HambzpY')
+ .setVideo('http://commondatastorage.googleapis.com/gtv-videos-bucket/sample/BigBuckBunny.mp4');
+ message.channel.send({
+ content: `${embed}`,
+ });
+ }
+});
+
+client.login('token');
diff --git a/examples/RichPresence.js b/examples/RichPresence.js
new file mode 100644
index 00000000..7238630
--- /dev/null
+++ b/examples/RichPresence.js
@@ -0,0 +1,46 @@
+const { Client, RichPresence, CustomStatus, SpotifyRPC } = require('discord.js-selfbot-v13');
+const client = new Client();
+
+client.on('ready', async () => {
+ console.log(`${client.user.username} is ready!`);
+ const getExtendURL = await RichPresence.getExternal(
+ client,
+ '367827983903490050',
+ 'https://assets.ppy.sh/beatmaps/1550633/covers/list.jpg', // Required if the image you use is not in Discord
+ );
+ const status = new RichPresence()
+ .setApplicationId('367827983903490050')
+ .setType('PLAYING')
+ .setURL('https://www.youtube.com/watch?v=5icFcPkVzMg')
+ .setState('Arcade Game')
+ .setName('osu!')
+ .setDetails('MariannE - Yooh')
+ .setParty({
+ max: 8,
+ current: 1,
+ })
+ .setStartTimestamp(Date.now())
+ .setAssetsLargeImage(getExtendURL[0].external_asset_path) // https://assets.ppy.sh/beatmaps/1550633/covers/list.jpg
+ .setAssetsLargeText('Idle')
+ .setAssetsSmallImage('373370493127884800') // https://discord.com/api/v9/oauth2/applications/367827983903490050/assets
+ .setAssetsSmallText('click the circles')
+ .addButton('Beatmap', 'https://osu.ppy.sh/beatmapsets/1391659#osu/2873429');
+ // Custom Status
+ const custom = new CustomStatus().setEmoji('π').setState('yum');
+ // Spotify
+ const spotify = new SpotifyRPC(client)
+ .setAssetsLargeImage('spotify:ab67616d00001e02768629f8bc5b39b68797d1bb') // Image ID
+ .setAssetsSmallImage('spotify:ab6761610000f178049d8aeae802c96c8208f3b7') // Image ID
+ .setAssetsLargeText('ζͺζ₯θΆε± (vol.1)') // Album Name
+ .setState('Yunomi; Kizuna AI') // Artists
+ .setDetails('γγγγγγΌγ') // Song name
+ .setStartTimestamp(Date.now())
+ .setEndTimestamp(Date.now() + 1_000 * (2 * 60 + 56)) // Song length = 2m56s
+ .setSongId('667eE4CFfNtJloC6Lvmgrx') // Song ID
+ .setAlbumId('6AAmvxoPoDbJAwbatKwMb9') // Album ID
+ .setArtistIds('2j00CVYTPx6q9ANbmB2keb', '2nKGmC5Mc13ct02xAY8ccS'); // Artist IDs
+
+ client.user.setPresence({ activities: [status, custom, spotify] });
+});
+
+client.login('token');
diff --git a/examples/SamsungRPC.js b/examples/SamsungRPC.js
new file mode 100644
index 00000000..ca61d88
--- /dev/null
+++ b/examples/SamsungRPC.js
@@ -0,0 +1,17 @@
+const { Client } = require('../src/index');
+
+const client = new Client();
+
+client.on('ready', async () => {
+ client.user.setSamsungActivity('com.YostarJP.BlueArchive', 'START');
+
+ setTimeout(() => {
+ client.user.setSamsungActivity('com.miHoYo.bh3oversea', 'UPDATE');
+ }, 30_000);
+
+ setTimeout(() => {
+ client.user.setSamsungActivity('com.miHoYo.GenshinImpact', 'STOP');
+ }, 60_000);
+});
+
+client.login('token');
diff --git a/examples/SlashCommand.md b/examples/SlashCommand.md
new file mode 100644
index 00000000..a0aeaf5
--- /dev/null
+++ b/examples/SlashCommand.md
@@ -0,0 +1,91 @@
+# Slash command
+
+```js
+TextBasedChannel.sendSlash(
+ user: BotId (Snowflake) | User (User.bot === true),
+ commandName: 'command_name [sub_group] [sub]',
+ ...args: (string|number|boolean|FileLike|undefined)[],
+): Promise | Modal>
+```
+
+## Basic
+
+### Demo
+
+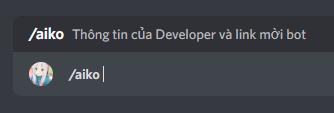
+
+### Code
+
+```js
+await channel.sendSlash('bot_id', 'aiko')
+```
+
+## Sub Command / Sub Group
+
+### Demo
+
+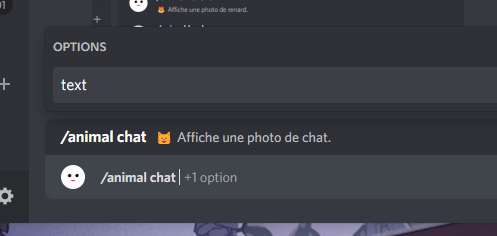
+
+### Code test
+
+```js
+await channel.sendSlash('450323683840491530', 'animal chat', 'bye')
+```
+
+## Attachment
+
+### Demo
+
+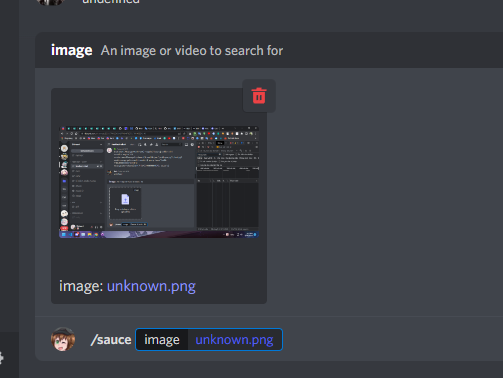
+
+### Code test
+
+```js
+const { MessageAttachment } = require('discord.js-selfbot-v13')
+const fs = require('fs')
+const a = new MessageAttachment(fs.readFileSync('./wallpaper.jpg') , 'test.jpg')
+await message.channel.sendSlash('718642000898818048', 'sauce', a)
+```
+
+### Result
+
+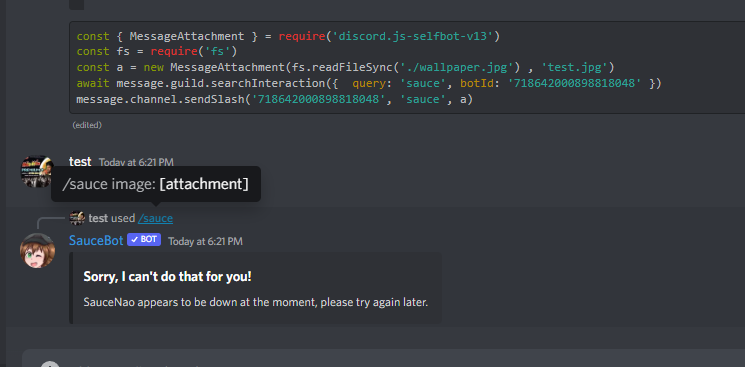
+
+## Skip options
+
+### Demo Command
+
+
+
+
+
+
+
+
+
+### Code
+```js
+ const channel = client.channels.cache.get('channel_id');
+ const response = await channel.sendSlash(
+ 'bot_id',
+ 'image make',
+ 'MeinaMix - v11',
+ 'Phone (9:16) [576x1024 | 810x1440]',
+ '2', // String choices, not number
+ undefined, // VAE
+ undefined, // sdxl_refiner
+ undefined, // sampling_method,
+ 30,
+ );
+ // Submit Modal
+ if (!response.isMessage) { // Modal
+ response.components[0].components[0].setValue(
+ '1girl, brown hair, green eyes, colorful, autumn, cumulonimbus clouds',
+ );
+ response.components[1].components[0].setValue(
+ '(worst quality:1.4), (low quality:1.4), (normal quality:1.4), (ugly:1.4), (bad anatomy:1.4), (extra limbs:1.2), (text, error, signature, watermark:1.2), (bad legs, incomplete legs), (bad feet), (bad arms), (bad hands, too many hands, mutated hands), (zombie, sketch, interlocked fingers, comic, morbid), cropped, long neck, lowres, missing fingers, missing arms, missing legs, extra fingers, extra digit, fewer digits, jpeg artifacts',
+ );
+ await response.reply();
+ }
+
+```
\ No newline at end of file
diff --git a/examples/VoiceMessage.js b/examples/VoiceMessage.js
new file mode 100644
index 00000000..cce1253
--- /dev/null
+++ b/examples/VoiceMessage.js
@@ -0,0 +1,21 @@
+const { Client, MessageAttachment } = require('../src/index');
+const client = new Client();
+
+client.on('ready', async () => {
+ console.log(`${client.user.username} is ready!`);
+ const channel = client.channels.cache.get('channel_id');
+ const attachment = new MessageAttachment(
+ './test.mp3', // path file
+ 'random_file_name.ogg', // must be .ogg
+ {
+ waveform: '=',
+ duration_secs: 1, // any number you want
+ },
+ );
+ channel.send({
+ files: [attachment],
+ flags: 'IS_VOICE_MESSAGE',
+ });
+});
+
+client.login('token');
diff --git a/src/structures/RichPresence.js b/src/structures/RichPresence.js
index b649211..ea1e787 100644
--- a/src/structures/RichPresence.js
+++ b/src/structures/RichPresence.js
@@ -180,9 +180,17 @@ class RichPresence {
this.buttons = data.buttons;
this.metadata = data.metadata;
}
+ /**
+ * @typedef {string} RichPresenceImage
+ * Support:
+ * - cdn.discordapp.com
+ * - media.discordapp.net
+ * - Asset ID (From https://discord.com/api/v9/oauth2/applications/:id/assets)
+ * - ExternalAssets (mp:external/)
+ */
/**
* Set the large image of this activity
- * @param {?any} image The large image asset's id
+ * @param {?RichPresenceImage} image The large image asset's id
* @returns {RichPresence}
*/
setAssetsLargeImage(image) {
@@ -198,14 +206,7 @@ class RichPresence {
.replace('http://media.discordapp.net/', 'mp:');
//
if (!image.startsWith('mp:') && !this.ipc) {
- throw new Error(
- 'INVALID_URL',
- `
-If you want to set the URL directly, it should be the Discord URL (cdn.discordapp.com | media.discordapp.net)
-Or follow these instructions:
-https://github.com/aiko-chan-ai/discord.js-selfbot-v13/blob/main/Documents/RichPresence.md#method-3-custom-url-2378
-`,
- );
+ throw new Error('INVALID_URL');
}
} else if (/^[0-9]{17,19}$/.test(image)) {
// ID Assets
@@ -219,7 +220,7 @@ https://github.com/aiko-chan-ai/discord.js-selfbot-v13/blob/main/Documents/RichP
}
/**
* Set the small image of this activity
- * @param {?any} image The small image asset's id
+ * @param {?RichPresenceImage} image The small image asset's id
* @returns {RichPresence}
*/
setAssetsSmallImage(image) {
@@ -235,14 +236,7 @@ https://github.com/aiko-chan-ai/discord.js-selfbot-v13/blob/main/Documents/RichP
.replace('http://media.discordapp.net/', 'mp:');
//
if (!image.startsWith('mp:') && !this.ipc) {
- throw new Error(
- 'INVALID_URL',
- `
-If you want to set the URL directly, it should be the Discord URL (cdn.discordapp.com | media.discordapp.net)
-Or follow these instructions:
-https://github.com/aiko-chan-ai/discord.js-selfbot-v13/blob/main/Documents/RichPresence.md#method-3-custom-url-2378
-`,
- );
+ throw new Error('INVALID_URL');
}
} else if (/^[0-9]{17,19}$/.test(image)) {
// ID Assets
diff --git a/src/structures/WebEmbed.js b/src/structures/WebEmbed.js
index 8576690..c0a8653 100644
--- a/src/structures/WebEmbed.js
+++ b/src/structures/WebEmbed.js
@@ -8,7 +8,11 @@ const Util = require('../util/Util');
/**
* Send Embedlink to Discord
- * Need to change WebEmbed API server (because heroku is no longer free)
+ * Only works with Discord Web and Discord Client (no custom theme installed)
+ * - No Timestamp, Footer, Fields, Author iconURL
+ * - Video with Embed working
+ * - Can only choose between image and thumbnail
+ * - Description limit 350 characters
*/
class WebEmbed {
/**
diff --git a/typings/index.d.ts b/typings/index.d.ts
index d1582f0..d73d255 100644
--- a/typings/index.d.ts
+++ b/typings/index.d.ts
@@ -191,9 +191,9 @@ export class RichPresence {
public type: ActivityType;
public url: string | null;
public ipc: boolean;
- public setAssetsLargeImage(image?: any): this;
+ public setAssetsLargeImage(image?: string): this;
public setAssetsLargeText(text?: string): this;
- public setAssetsSmallImage(image?: any): this;
+ public setAssetsSmallImage(image?: string): this;
public setAssetsSmallText(text?: string): this;
public setName(name?: string): this;
public setURL(url?: string): this;