diff --git a/DOCUMENT.md b/DOCUMENT.md
index 1d524e0..b237daa 100644
--- a/DOCUMENT.md
+++ b/DOCUMENT.md
@@ -119,6 +119,7 @@ User {
flags: UserFlagsBitField { bitfield: 256 },
friend: false,
blocked: false,
+ note: null,
connectedAccounds: [],
premiumSince: 1623357181151,
premiumGuildSince: 0,
@@ -303,14 +304,35 @@ await message.contextMenu(botID, commandName);
- Credit: [Here](https://www.reddit.com/r/Discord_selfbots/comments/tczprx/discum_help_creating_a_selfbot_that_can_do_ping/)
-## User HypeSquad
+## User & ClientUser Method
Click to show
```js
+// HypeSquad
await client.user.setHypeSquad('HOUSE_BRAVERY');
await client.user.setHypeSquad('HOUSE_BRILLIANCE');
await client.user.setHypeSquad('HOUSE_BALANCE');
+// Set Note to User
+await user.setNote('Hello World');
+// Set Username
+await client.user.setUsername('new username', 'password');
+// Set Accent Color
+await client.user.setAccentColor('RED'); // set color same as Embed.setColor()
+// Set Banner
+await client.user.setBanner('image file / image url'); // same as setAvatar & Require Nitro level 2
+// Set Discord Tag
+await client.user.setDiscriminator('1234', 'password'); // #1234
+// Set About me
+await client.user.setAboutMe('Hello World');
+// Set Email
+await client.user.setEmail('aiko.dev@mail.nezukobot.vn', 'password'); // It is clone email =))
+// Change Password
+await client.user.setPassword('old password', 'new password');
+// Disable Account
+await client.user.disableAccount('password');
+// Delete Account [WARNING] Cannot be changed once used!
+await client.user.deleteAccount('password');
```
diff --git a/README.md b/README.md
index dd962ab..6aac130 100644
--- a/README.md
+++ b/README.md
@@ -60,13 +60,13 @@ window.webpackChunkdiscord_app.push([[Math.random()], {}, (req) => {for (const m
Credit:
[hxr404](https://github.com/hxr404/Discord-Console-hacks)
## Selfbot feature ?
-- Friends and Block Members
-- Discord Apps Setting [Theme, Language, HypeSquad, etc.]
-- Get Profile GuildMember [Nitro Time, Boost Time, Connected Account, Bio, etc.]
-- Setting Position Guild and Folder
-- Custom Status and RPC (without button, because it's not working)
-- Interaction [Button, MessageSelectMenu, Slash, ContextMenu]
-- You can request more features for my module by placing an issue!
+- [Friends and Block Members](https://github.com/aiko-chan-ai/discord.js-selfbot-v13/blob/main/DOCUMENT.md#discord-user-friend--blocked)
+- [Discord Apps Setting: Theme, Language, HypeSquad, etc.](https://github.com/aiko-chan-ai/discord.js-selfbot-v13/blob/main/DOCUMENT.md#user-settings)
+- [Get Profile GuildMember: Nitro Time, Boost Time, Connected Account, Bio, etc.](https://github.com/aiko-chan-ai/discord.js-selfbot-v13/blob/main/DOCUMENT.md#discord-user-info)
+- [Setting Position Guild and Folder](https://github.com/aiko-chan-ai/discord.js-selfbot-v13/blob/main/DOCUMENT.md#discord-guild-set-position)
+- [Custom Status and RPC (without button, because it's not working)](https://github.com/aiko-chan-ai/discord.js-selfbot-v13/blob/main/DOCUMENT.md#custom-status-and-rpc)
+- [Interaction: Button, MessageSelectMenu, Slash, ContextMenu](https://github.com/aiko-chan-ai/discord.js-selfbot-v13/blob/main/DOCUMENT.md#interaction)
+- [You can request more features for my module by placing an issue!](https://github.com/aiko-chan-ai/discord.js-selfbot-v13/issues)
- Click [here](https://github.com/aiko-chan-ai/discord.js-selfbot-v13/blob/main/DOCUMENT.md) to see the patched functions
## Links [Discord.js]
@@ -88,5 +88,13 @@ Credit: 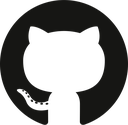
Vietnamese
- Tóm lại là module này dùng Discord.js v13 , API v10 nên chưa chết sớm đâu, cứ dùng đi =))
\ No newline at end of file
diff --git a/package.json b/package.json
index 7fcb4c5..11f721f 100644
--- a/package.json
+++ b/package.json
@@ -1,6 +1,6 @@
{
"name": "discord.js-selfbot-v13",
- "version": "1.1.7",
+ "version": "1.2.0",
"description": "A unofficial discord.js fork for creating selfbots [Based on discord.js v13]",
"main": "./src/index.js",
"types": "./typings/index.d.ts",
diff --git a/src/client/Client.js b/src/client/Client.js
index 6635923..3cbccdf 100644
--- a/src/client/Client.js
+++ b/src/client/Client.js
@@ -193,6 +193,12 @@ class Client extends BaseClient {
*/
this.readyAt = null;
+ /**
+ * Password cache
+ * @type {?string}
+ */
+ this.password = null;
+
if (this.options.messageSweepInterval > 0) {
process.emitWarning(
'The message sweeping client options are deprecated, use the global sweepers instead.',
diff --git a/src/structures/ClientUser.js b/src/structures/ClientUser.js
index c6297d3..5f5374c 100644
--- a/src/structures/ClientUser.js
+++ b/src/structures/ClientUser.js
@@ -3,212 +3,350 @@
const User = require('./User');
const DataResolver = require('../util/DataResolver');
const { HypeSquadOptions } = require('../util/Constants');
+const { Util } = require('..');
/**
* Represents the logged in client's Discord user.
* @extends {User}
*/
class ClientUser extends User {
- _patch(data) {
- super._patch(data);
+ _patch(data) {
+ super._patch(data);
- if ('verified' in data) {
- /**
- * Whether or not this account has been verified
- * @type {boolean}
- */
- this.verified = data.verified;
- }
+ if ('verified' in data) {
+ /**
+ * Whether or not this account has been verified
+ * @type {boolean}
+ */
+ this.verified = data.verified;
+ }
- if ('mfa_enabled' in data) {
- /**
- * If the bot's {@link ClientApplication#owner Owner} has MFA enabled on their account
- * @type {?boolean}
- */
- this.mfaEnabled = typeof data.mfa_enabled === 'boolean' ? data.mfa_enabled : null;
- } else {
- this.mfaEnabled ??= null;
- }
+ if ('mfa_enabled' in data) {
+ /**
+ * If the bot's {@link ClientApplication#owner Owner} has MFA enabled on their account
+ * @type {?boolean}
+ */
+ this.mfaEnabled =
+ typeof data.mfa_enabled === 'boolean' ? data.mfa_enabled : null;
+ } else {
+ this.mfaEnabled ??= null;
+ }
- if ('token' in data) this.client.token = data.token;
+ if ('token' in data) this.client.token = data.token;
- // Add (Selfbot)
- if ('premium' in data) this.nitro = data.premium;
- /**
- * Nitro Status
- * `0`: None
- * `1`: Classic
- * `2`: Boost
- * @external
- * https://discord.com/developers/docs/resources/user#user-object-premium-types
- * @type {Number}
- */
- if ('purchased_flags' in data) this.nitroType = data.purchased_flags;
- if ('phone' in data) this.phoneNumber = data.phone;
- if ('nsfw_allowed' in data) this.nsfwAllowed = data.nsfw_allowed;
- if ('email' in data) this.emailAddress = data.email;
- }
+ // Add (Selfbot)
+ if ('premium' in data) this.nitro = data.premium;
+ /**
+ * Nitro Status
+ * `0`: None
+ * `1`: Classic
+ * `2`: Boost
+ * @external
+ * https://discord.com/developers/docs/resources/user#user-object-premium-types
+ * @type {Number}
+ */
+ if ('purchased_flags' in data) this.nitroType = data.purchased_flags;
+ if ('phone' in data) this.phoneNumber = data.phone;
+ if ('nsfw_allowed' in data) this.nsfwAllowed = data.nsfw_allowed;
+ if ('email' in data) this.emailAddress = data.email;
+ }
- /**
- * Represents the client user's presence
- * @type {ClientPresence}
- * @readonly
- */
- get presence() {
- return this.client.presence;
- }
+ /**
+ * Represents the client user's presence
+ * @type {ClientPresence}
+ * @readonly
+ */
+ get presence() {
+ return this.client.presence;
+ }
- /**
- * Data used to edit the logged in client
- * @typedef {Object} ClientUserEditData
- * @property {string} [username] The new username
- * @property {?(BufferResolvable|Base64Resolvable)} [avatar] The new avatar
- */
+ /**
+ * Data used to edit the logged in client
+ * @typedef {Object} ClientUserEditData
+ * @property {string} [username] The new username
+ * @property {?(BufferResolvable|Base64Resolvable)} [avatar] The new avatar
+ */
- /**
- * Edits the logged in client.
- * @param {ClientUserEditData} data The new data
- * @returns {Promise}
- */
- async edit(data) {
- if (typeof data.avatar !== 'undefined') data.avatar = await DataResolver.resolveImage(data.avatar);
- const newData = await this.client.api.users('@me').patch({ data });
- this.client.token = newData.token;
- const { updated } = this.client.actions.UserUpdate.handle(newData);
- return updated ?? this;
- }
+ /**
+ * Edits the logged in client.
+ * @param {ClientUserEditData} data The new data
+ * @returns {Promise}
+ */
+ async edit(data) {
+ if (typeof data.avatar !== 'undefined')
+ data.avatar = await DataResolver.resolveImage(data.avatar);
+ if (typeof data.banner !== 'undefined')
+ data.banner = await DataResolver.resolveImage(data.banner);
+ const newData = await this.client.api.users('@me').patch({ data });
+ this.client.token = newData.token;
+ this.client.password = data?.password
+ ? data?.password
+ : this.client.password;
+ const { updated } = this.client.actions.UserUpdate.handle(newData);
+ return updated ?? this;
+ }
- /**
- * Sets the username of the logged in client.
- * Changing usernames in Discord is heavily rate limited, with only 2 requests
- * every hour. Use this sparingly!
- * @param {string} username The new username
- * @returns {Promise}
- * @example
- * // Set username
- * client.user.setUsername('discordjs')
- * .then(user => console.log(`My new username is ${user.username}`))
- * .catch(console.error);
- */
- setUsername(username) {
- return this.edit({ username });
- }
+ /**
+ * Sets the username of the logged in client.
+ * Changing usernames in Discord is heavily rate limited, with only 2 requests
+ * every hour. Use this sparingly!
+ * @param {string} username The new username
+ * @param {string} password The password of the account
+ * @returns {Promise}
+ * @example
+ * // Set username
+ * client.user.setUsername('discordjs')
+ * .then(user => console.log(`My new username is ${user.username}`))
+ * .catch(console.error);
+ */
+ setUsername(username, password) {
+ if (!password && !this.client.password)
+ throw new Error('A password is required to change a username.');
+ return this.edit({
+ username,
+ password: this.client.password ? this.client.password : password,
+ });
+ }
- /**
- * Sets the avatar of the logged in client.
- * @param {?(BufferResolvable|Base64Resolvable)} avatar The new avatar
- * @returns {Promise}
- * @example
- * // Set avatar
- * client.user.setAvatar('./avatar.png')
- * .then(user => console.log(`New avatar set!`))
- * .catch(console.error);
- */
- setAvatar(avatar) {
- return this.edit({ avatar });
- }
- /**
- * Set HyperSquad House
- * @param {HypeSquadOptions} type
- * `HOUSE_BRAVERY`: 1
- * `HOUSE_BRILLIANCE`: 2
- * `HOUSE_BALANCE`: 3
- * @returns {Promise}
- * @example
- * // Set HyperSquad HOUSE_BRAVERY
- * client.user.setHypeSquad(1); || client.user.setHypeSquad('HOUSE_BRAVERY');
- */
- async setHypeSquad(type) {
- const id = typeof type === 'string' ? HypeSquadOptions[type] : type;
- if(!id) throw new Error('Invalid HypeSquad type.');
- return await this.client.api.hypesquad.online.post({ data: {house_id: id} });
- }
+ /**
+ * Sets the avatar of the logged in client.
+ * @param {?(BufferResolvable|Base64Resolvable)} avatar The new avatar
+ * @returns {Promise}
+ * @example
+ * // Set avatar
+ * client.user.setAvatar('./avatar.png')
+ * .then(user => console.log(`New avatar set!`))
+ * .catch(console.error);
+ */
+ setAvatar(avatar) {
+ return this.edit({ avatar });
+ }
+ /**
+ * Sets the banner of the logged in client.
+ * @param {?(BufferResolvable|Base64Resolvable)} banner The new banner
+ * @returns {Promise}
+ * @example
+ * // Set banner
+ * client.user.setBanner('./banner.png')
+ * .then(user => console.log(`New banner set!`))
+ * .catch(console.error);
+ */
+ setBanner(banner) {
+ if (this.nitroType !== 2)
+ throw new Error(
+ 'You must be a Nitro Boosted User to change your banner.',
+ );
+ return this.edit({ banner });
+ }
- /**
- * Options for setting activities
- * @typedef {Object} ActivitiesOptions
- * @property {string} [name] Name of the activity
- * @property {ActivityType|number} [type] Type of the activity
- * @property {string} [url] Twitch / YouTube stream URL
- */
+ /**
+ * Set HyperSquad House
+ * @param {HypeSquadOptions} type
+ * `HOUSE_BRAVERY`: 1
+ * `HOUSE_BRILLIANCE`: 2
+ * `HOUSE_BALANCE`: 3
+ * @returns {Promise}
+ * @example
+ * // Set HyperSquad HOUSE_BRAVERY
+ * client.user.setHypeSquad(1); || client.user.setHypeSquad('HOUSE_BRAVERY');
+ */
+ async setHypeSquad(type) {
+ const id = typeof type === 'string' ? HypeSquadOptions[type] : type;
+ if (!id) throw new Error('Invalid HypeSquad type.');
+ return await this.client.api.hypesquad.online.post({
+ data: { house_id: id },
+ });
+ }
- /**
- * Data resembling a raw Discord presence.
- * @typedef {Object} PresenceData
- * @property {PresenceStatusData} [status] Status of the user
- * @property {boolean} [afk] Whether the user is AFK
- * @property {ActivitiesOptions[]} [activities] Activity the user is playing
- * @property {number|number[]} [shardId] Shard id(s) to have the activity set on
- */
+ /**
+ * Set Accent color
+ * @param {ColorResolvable} color Color to set
+ * @returns {Promise}
+ */
+ setAccentColor(color = null) {
+ return this.edit({ accent_color: color ? Util.resolveColor(color) : null });
+ }
- /**
- * Sets the full presence of the client user.
- * @param {PresenceData} data Data for the presence
- * @returns {ClientPresence}
- * @example
- * // Set the client user's presence
- * client.user.setPresence({ activities: [{ name: 'with discord.js' }], status: 'idle' });
- */
- setPresence(data) {
- return this.client.presence.set(data);
- }
+ /**
+ * Set discriminator
+ * @param {User.discriminator} discriminator It is #1234
+ * @param {string} password The password of the account
+ * @returns {Promise}
+ */
+ setDiscriminator(discriminator, password) {
+ if (!password && !this.client.password)
+ throw new Error('A password is required to change a discriminator.');
+ return this.edit({
+ discriminator,
+ password: this.client.password ? this.client.password : password,
+ });
+ }
- /**
- * A user's status. Must be one of:
- * * `online`
- * * `idle`
- * * `invisible`
- * * `dnd` (do not disturb)
- * @typedef {string} PresenceStatusData
- */
+ /**
+ * Set About me
+ * @param {String} bio Bio to set
+ * @returns {Promise}
+ */
+ setAboutMe(bio = null) {
+ return this.edit({
+ bio,
+ });
+ }
- /**
- * Sets the status of the client user.
- * @param {PresenceStatusData} status Status to change to
- * @param {number|number[]} [shardId] Shard id(s) to have the activity set on
- * @returns {ClientPresence}
- * @example
- * // Set the client user's status
- * client.user.setStatus('idle');
- */
- setStatus(status, shardId) {
- return this.setPresence({ status, shardId });
- }
+ /**
+ * Change the email
+ * @param {Email} email Email to change
+ * @param {string} password Password of the account
+ * @returns {Promise}
+ */
+ setEmail(email, password) {
+ if (!password && !this.client.password)
+ throw new Error('A password is required to change a email.');
+ return this.edit({
+ email,
+ password: this.client.password ? this.client.password : password,
+ });
+ }
- /**
- * Options for setting an activity.
- * @typedef {Object} ActivityOptions
- * @property {string} [name] Name of the activity
- * @property {string} [url] Twitch / YouTube stream URL
- * @property {ActivityType|number} [type] Type of the activity
- * @property {number|number[]} [shardId] Shard Id(s) to have the activity set on
- */
+ /**
+ * Set new password
+ * @param {string} oldPassword Old password
+ * @param {string} newPassword New password to set
+ * @returns {Promise}
+ */
+ setPassword(oldPassword, newPassword) {
+ if (!oldPassword && !this.client.password)
+ throw new Error('A password is required to change a password.');
+ if (!newPassword) throw new Error('New password is required.');
+ return this.edit({
+ password: this.client.password ? this.client.password : oldPassword,
+ new_password: newPassword,
+ });
+ }
- /**
- * Sets the activity the client user is playing.
- * @param {string|ActivityOptions} [name] Activity being played, or options for setting the activity
- * @param {ActivityOptions} [options] Options for setting the activity
- * @returns {ClientPresence}
- * @example
- * // Set the client user's activity
- * client.user.setActivity('discord.js', { type: 'WATCHING' });
- */
- setActivity(name, options = {}) {
- if (!name) return this.setPresence({ activities: [], shardId: options.shardId });
+ /**
+ * Disable account
+ * @param {string} password Password of the account
+ * @returns {Promise}
+ */
+ async disableAccount(password) {
+ if (!password && !this.client.password)
+ throw new Error('A password is required to disable an account.');
+ return await this.client.api.users['@me'].disable.post({
+ data: {
+ password: this.client.password ? this.client.password : password,
+ },
+ });
+ }
- const activity = Object.assign({}, options, typeof name === 'object' ? name : { name });
- return this.setPresence({ activities: [activity], shardId: activity.shardId });
- }
+ /**
+ * Delete account. Warning: Cannot be changed once used!
+ * @param {string} password Password of the account
+ * @returns {Promise}
+ */
+ async deleteAccount(password) {
+ if (!password && !this.client.password)
+ throw new Error('A password is required to delete an account.');
+ return await this.client.api.users['@me'].delete.post({
+ data: {
+ password: this.client.password ? this.client.password : password,
+ },
+ });
+ }
- /**
- * Sets/removes the AFK flag for the client user.
- * @param {boolean} [afk=true] Whether or not the user is AFK
- * @param {number|number[]} [shardId] Shard Id(s) to have the AFK flag set on
- * @returns {ClientPresence}
- */
- setAFK(afk = true, shardId) {
- return this.setPresence({ afk, shardId });
- }
+ /**
+ * Options for setting activities
+ * @typedef {Object} ActivitiesOptions
+ * @property {string} [name] Name of the activity
+ * @property {ActivityType|number} [type] Type of the activity
+ * @property {string} [url] Twitch / YouTube stream URL
+ */
+
+ /**
+ * Data resembling a raw Discord presence.
+ * @typedef {Object} PresenceData
+ * @property {PresenceStatusData} [status] Status of the user
+ * @property {boolean} [afk] Whether the user is AFK
+ * @property {ActivitiesOptions[]} [activities] Activity the user is playing
+ * @property {number|number[]} [shardId] Shard id(s) to have the activity set on
+ */
+
+ /**
+ * Sets the full presence of the client user.
+ * @param {PresenceData} data Data for the presence
+ * @returns {ClientPresence}
+ * @example
+ * // Set the client user's presence
+ * client.user.setPresence({ activities: [{ name: 'with discord.js' }], status: 'idle' });
+ */
+ setPresence(data) {
+ return this.client.presence.set(data);
+ }
+
+ /**
+ * A user's status. Must be one of:
+ * * `online`
+ * * `idle`
+ * * `invisible`
+ * * `dnd` (do not disturb)
+ * @typedef {string} PresenceStatusData
+ */
+
+ /**
+ * Sets the status of the client user.
+ * @param {PresenceStatusData} status Status to change to
+ * @param {number|number[]} [shardId] Shard id(s) to have the activity set on
+ * @returns {ClientPresence}
+ * @example
+ * // Set the client user's status
+ * client.user.setStatus('idle');
+ */
+ setStatus(status, shardId) {
+ return this.setPresence({ status, shardId });
+ }
+
+ /**
+ * Options for setting an activity.
+ * @typedef {Object} ActivityOptions
+ * @property {string} [name] Name of the activity
+ * @property {string} [url] Twitch / YouTube stream URL
+ * @property {ActivityType|number} [type] Type of the activity
+ * @property {number|number[]} [shardId] Shard Id(s) to have the activity set on
+ */
+
+ /**
+ * Sets the activity the client user is playing.
+ * @param {string|ActivityOptions} [name] Activity being played, or options for setting the activity
+ * @param {ActivityOptions} [options] Options for setting the activity
+ * @returns {ClientPresence}
+ * @example
+ * // Set the client user's activity
+ * client.user.setActivity('discord.js', { type: 'WATCHING' });
+ */
+ setActivity(name, options = {}) {
+ if (!name)
+ return this.setPresence({ activities: [], shardId: options.shardId });
+
+ const activity = Object.assign(
+ {},
+ options,
+ typeof name === 'object' ? name : { name },
+ );
+ return this.setPresence({
+ activities: [activity],
+ shardId: activity.shardId,
+ });
+ }
+
+ /**
+ * Sets/removes the AFK flag for the client user.
+ * @param {boolean} [afk=true] Whether or not the user is AFK
+ * @param {number|number[]} [shardId] Shard Id(s) to have the AFK flag set on
+ * @returns {ClientPresence}
+ */
+ setAFK(afk = true, shardId) {
+ return this.setPresence({ afk, shardId });
+ }
}
module.exports = ClientUser;
diff --git a/src/structures/User.js b/src/structures/User.js
index b3717a1..491a9c8 100644
--- a/src/structures/User.js
+++ b/src/structures/User.js
@@ -423,6 +423,11 @@ class User extends Base {
return json;
}
+ /**
+ * Set note to user
+ * @param {String} note Note to set
+ * @returns {Promise}
+ */
async setNote(note = null) {
await this.client.api.users['@me']
.notes(id)
diff --git a/typings/index.d.ts b/typings/index.d.ts
index c5e761a..f443e2e 100644
--- a/typings/index.d.ts
+++ b/typings/index.d.ts
@@ -565,6 +565,7 @@ export class Client extends BaseClient {
public users: UserManager;
public voice: ClientVoiceManager;
public ws: WebSocketManager;
+ public password: String | null;
public destroy(): void;
public fetchGuildPreview(guild: GuildResolvable): Promise;
public fetchInvite(invite: InviteResolvable, options?: ClientFetchInviteOptions): Promise;
@@ -636,10 +637,19 @@ export class ClientUser extends User {
public setActivity(name: string, options?: ActivityOptions): ClientPresence;
public setAFK(afk?: boolean, shardId?: number | number[]): ClientPresence;
public setAvatar(avatar: BufferResolvable | Base64Resolvable | null): Promise;
+ public setBanner(banner: BufferResolvable | Base64Resolvable | null): Promise;
public setPresence(data: PresenceData): ClientPresence;
public setStatus(status: PresenceStatusData, shardId?: number | number[]): ClientPresence;
- public setUsername(username: string): Promise;
+ public setUsername(username: string, password: string): Promise;
public setHypeSquad(type: HypeSquadOptions): Promise;
+ public setAccentColor(color: ColorResolvable): Promise;
+ public setDiscriminator(discriminator: string, password: string): Promise;
+ public setAboutMe(bio: string): Promise;
+ public setBanner(): Promise;
+ public setEmail(email: string, password: string): Promise;
+ public setPassword(oldPassword: string, newPassword: string): Promise;
+ public disableAccount(password: string): Promise;
+ public deleteAccount(password: string): Promise;
// Selfbot
public readonly nitro: boolean;
/**
@@ -2427,6 +2437,7 @@ export class User extends PartialTextBasedChannel(Base) {
public system: boolean;
public readonly tag: string;
public username: string;
+ public note: string | null;
public avatarURL(options?: ImageURLOptions): string | null;
public bannerURL(options?: ImageURLOptions): string | null;
public createDM(force?: boolean): Promise;
@@ -2440,6 +2451,7 @@ export class User extends PartialTextBasedChannel(Base) {
public sendFriendRequest(): Promise;
public unFriend(): Promise;
public unBlock(): Promise;
+ public setNote(note): Promise;
public getProfile(): Promise;
public toString(): UserMention;
}