refactor(RichPresence): for Selfbot
This commit is contained in:
parent
0bb0b0e59d
commit
fd17a6a6f0
@ -156,38 +156,44 @@ client.user.setActivity(custom);
|
||||
```
|
||||
|
||||
Rich Presence [Custom]
|
||||
Usage: see this module https://www.npmjs.com/package/discordrpcgenerator
|
||||
```js
|
||||
const RPC = require('discord-rpc-contructor');
|
||||
const r = new RPC.Rpc()
|
||||
const r = new Discord.RichPresence()
|
||||
.setApplicationId('817229550684471297')
|
||||
.setType(0)
|
||||
.setType('STREAMING')
|
||||
.setURL('https://youtube.com/watch?v=dQw4w9WgXcQ')
|
||||
.setState('State')
|
||||
.setName('Name')
|
||||
.setDetails('Details')
|
||||
.setParty({
|
||||
size: [1, 2],
|
||||
id: RPC.uuid(),
|
||||
max: 9,
|
||||
current: 1,
|
||||
id: Discord.getUUID(),
|
||||
})
|
||||
.setStartTimestamp(Date.now())
|
||||
.setAssetsLargeImage('929325841350000660')
|
||||
.setAssetsLargeText('Youtube')
|
||||
.setAssetsSmallImage('895316294222635008')
|
||||
.setAssetsSmallText('Bot')
|
||||
client.user.setActivity(r.toDiscord().game);
|
||||
// Button not working
|
||||
.addButton('name', 'https://link.com/')
|
||||
client.user.setActivity(r.toJSON());
|
||||
```
|
||||
<img src='https://cdn.discordapp.com/attachments/820557032016969751/955767445220646922/unknown.png'>
|
||||
<img src='https://cdn.discordapp.com/attachments/820557032016969751/994300662378676264/unknown.png'>
|
||||
|
||||
Rich Presence with Twitch / Spotify
|
||||
Rich Presence with Spotify
|
||||
```js
|
||||
Update soon ~
|
||||
const r = new Discord.SpotifyRPC(client)
|
||||
.setAssetsLargeImage("spotify:ab67616d00001e02768629f8bc5b39b68797d1bb") // Image ID
|
||||
.setAssetsSmallImage("spotify:ab67616d00001e02768629f8bc5b39b68797d1bb")
|
||||
.setState('state')
|
||||
.setDetails('details')
|
||||
.setSongId('667eE4CFfNtJloC6Lvmgrx'); // Song ID
|
||||
client.user.setActivity(r.toJSON());
|
||||
```
|
||||
<img src='https://cdn.discordapp.com/attachments/820557032016969751/994308354535075980/unknown.png'>
|
||||
|
||||
|
||||
<strong>New: You can now add custom images for RPC !</strong>
|
||||
> Tutorial:
|
||||
|
||||
<strong>Method 1</strong>
|
||||
|
||||
+ Step 1: Send photos by embed.thumbnail
|
||||
|
||||
@ -203,67 +209,27 @@ const proxyURL = msg.embeds[0].thumbnail.proxyURL;
|
||||
+ Step 3: Put the URL in the constructor
|
||||
|
||||
```js
|
||||
const RPC = require('discord-rpc-contructor');
|
||||
const r = new RPC.Rpc()
|
||||
const r = new Discord.RichPresence()
|
||||
.setApplicationId('817229550684471297')
|
||||
.setType(0)
|
||||
.setType('STREAMING')
|
||||
.setURL('https://youtube.com/watch?v=dQw4w9WgXcQ')
|
||||
.setState('State')
|
||||
.setName('Name')
|
||||
.setDetails('Details')
|
||||
.setAssetsLargeImage(proxyURL) // Custom image
|
||||
.setParty({
|
||||
max: 9,
|
||||
current: 1,
|
||||
id: Discord.getUUID(),
|
||||
})
|
||||
.setStartTimestamp(Date.now())
|
||||
.setAssetsLargeImage(proxyURL)
|
||||
.setAssetsLargeText('Youtube')
|
||||
.setAssetsSmallImage('895316294222635008')
|
||||
.setAssetsSmallText('Bot')
|
||||
.addButton('name', 'https://link.com/')
|
||||
client.user.setActivity(r.toDiscord().game);
|
||||
```
|
||||
|
||||
<strong>Method 2 - require ver 1.1.1 - latest</strong>
|
||||
|
||||
```
|
||||
Note: If you use time-varying RPC, or if it takes too long to place images, use the 2nd method because it spams me too much .-.
|
||||
```
|
||||
|
||||
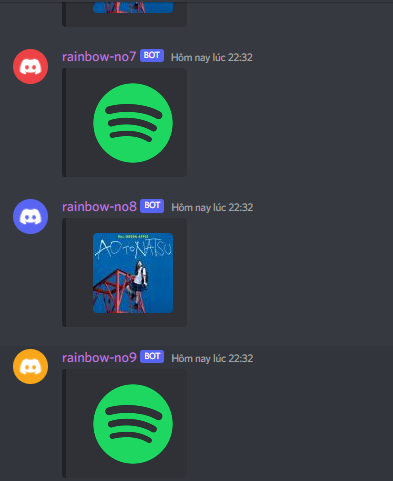
|
||||
|
||||
|
||||
```js
|
||||
const RPC = require('discord-rpc-contructor');
|
||||
const r = new RPC.Rpc()
|
||||
.setApplicationId('817229550684471297')
|
||||
.setType(0)
|
||||
.setState('State')
|
||||
.setName('Name')
|
||||
.setDetails('Details')
|
||||
.setAssetsLargeImage(await RPC.getImageCustom(imageURL1)) // Custom image
|
||||
.setAssetsLargeText('Youtube')
|
||||
.setAssetsSmallImage(await RPC.getImageCustom(imageURL2)) // Custom image
|
||||
.setAssetsSmallText('Bot')
|
||||
client.user.setActivity(r.toDiscord().game);
|
||||
```
|
||||
|
||||
|
||||
<strong>How to get AssetID ?</strong>
|
||||
|
||||
Code
|
||||
|
||||
```js
|
||||
const RPC = require('discord-rpc-contructor');
|
||||
// Bot ID
|
||||
RPC.getRpcImages('817229550684471297').then(console.log);
|
||||
```
|
||||
Return
|
||||
```js
|
||||
// ID is AssetID
|
||||
[
|
||||
{ id: '838629816881381376', type: 1, name: 'honkai' },
|
||||
{ id: '853533658250084352', type: 1, name: 'vscode' },
|
||||
{ id: '895316294222635008', type: 1, name: 'botsagiri' },
|
||||
{ id: '929324633063292929', type: 1, name: 'soundcloud' },
|
||||
{ id: '929324634858479666', type: 1, name: 'spotify' },
|
||||
{ id: '929325841350000660', type: 1, name: 'youtube' }
|
||||
]
|
||||
```
|
||||
You can cache to use these files, do not run this function too much because it will be rate limit
|
||||
And you can change the status 5 times every 20 seconds!
|
||||
</details>
|
||||
|
||||
|
File diff suppressed because one or more lines are too long
29
package-lock.json
generated
29
package-lock.json
generated
@ -1,12 +1,12 @@
|
||||
{
|
||||
"name": "discord.js-selfbot-v13",
|
||||
"version": "2.3.62",
|
||||
"version": "2.3.63",
|
||||
"lockfileVersion": 2,
|
||||
"requires": true,
|
||||
"packages": {
|
||||
"": {
|
||||
"name": "discord.js-selfbot-v13",
|
||||
"version": "2.3.62",
|
||||
"version": "2.3.63",
|
||||
"license": "GNU General Public License v3.0",
|
||||
"dependencies": {
|
||||
"@aikochan2k6/qrcode-terminal": "^0.12.0",
|
||||
@ -21,7 +21,6 @@
|
||||
"chalk": "^4.1.2",
|
||||
"discord-api-types": "^0.36.0",
|
||||
"discord-bettermarkdown": "^1.2.0",
|
||||
"discord-rpc-contructor": "^1.1.5",
|
||||
"form-data": "^4.0.0",
|
||||
"json-bigint": "^1.0.0",
|
||||
"node-fetch": "^2.6.1",
|
||||
@ -4442,15 +4441,6 @@
|
||||
"discord.js": "^13.6.0"
|
||||
}
|
||||
},
|
||||
"node_modules/discord-rpc-contructor": {
|
||||
"version": "1.1.5",
|
||||
"resolved": "https://registry.npmjs.org/discord-rpc-contructor/-/discord-rpc-contructor-1.1.5.tgz",
|
||||
"integrity": "sha512-qMwlQXPRT5DoXXRXn3RqHfH28HV7bUOKhPEBS/BelUj5fUFKG9ZotwJ4NeAxk46SRtjz39kIDybomXfLV8vi2A==",
|
||||
"dependencies": {
|
||||
"lodash": "^4.17.21",
|
||||
"node-fetch": "^2.6.7"
|
||||
}
|
||||
},
|
||||
"node_modules/discord.js": {
|
||||
"version": "13.8.0",
|
||||
"resolved": "https://registry.npmjs.org/discord.js/-/discord.js-13.8.0.tgz",
|
||||
@ -8542,7 +8532,8 @@
|
||||
"node_modules/lodash": {
|
||||
"version": "4.17.21",
|
||||
"resolved": "https://registry.npmjs.org/lodash/-/lodash-4.17.21.tgz",
|
||||
"integrity": "sha512-v2kDEe57lecTulaDIuNTPy3Ry4gLGJ6Z1O3vE1krgXZNrsQ+LFTGHVxVjcXPs17LhbZVGedAJv8XZ1tvj5FvSg=="
|
||||
"integrity": "sha512-v2kDEe57lecTulaDIuNTPy3Ry4gLGJ6Z1O3vE1krgXZNrsQ+LFTGHVxVjcXPs17LhbZVGedAJv8XZ1tvj5FvSg==",
|
||||
"dev": true
|
||||
},
|
||||
"node_modules/lodash.camelcase": {
|
||||
"version": "4.3.0",
|
||||
@ -15954,15 +15945,6 @@
|
||||
"discord.js": "^13.6.0"
|
||||
}
|
||||
},
|
||||
"discord-rpc-contructor": {
|
||||
"version": "1.1.5",
|
||||
"resolved": "https://registry.npmjs.org/discord-rpc-contructor/-/discord-rpc-contructor-1.1.5.tgz",
|
||||
"integrity": "sha512-qMwlQXPRT5DoXXRXn3RqHfH28HV7bUOKhPEBS/BelUj5fUFKG9ZotwJ4NeAxk46SRtjz39kIDybomXfLV8vi2A==",
|
||||
"requires": {
|
||||
"lodash": "^4.17.21",
|
||||
"node-fetch": "^2.6.7"
|
||||
}
|
||||
},
|
||||
"discord.js": {
|
||||
"version": "13.8.0",
|
||||
"resolved": "https://registry.npmjs.org/discord.js/-/discord.js-13.8.0.tgz",
|
||||
@ -19095,7 +19077,8 @@
|
||||
"lodash": {
|
||||
"version": "4.17.21",
|
||||
"resolved": "https://registry.npmjs.org/lodash/-/lodash-4.17.21.tgz",
|
||||
"integrity": "sha512-v2kDEe57lecTulaDIuNTPy3Ry4gLGJ6Z1O3vE1krgXZNrsQ+LFTGHVxVjcXPs17LhbZVGedAJv8XZ1tvj5FvSg=="
|
||||
"integrity": "sha512-v2kDEe57lecTulaDIuNTPy3Ry4gLGJ6Z1O3vE1krgXZNrsQ+LFTGHVxVjcXPs17LhbZVGedAJv8XZ1tvj5FvSg==",
|
||||
"dev": true
|
||||
},
|
||||
"lodash.camelcase": {
|
||||
"version": "4.3.0",
|
||||
|
@ -1,6 +1,6 @@
|
||||
{
|
||||
"name": "discord.js-selfbot-v13",
|
||||
"version": "2.3.62",
|
||||
"version": "2.3.63",
|
||||
"description": "A unofficial discord.js fork for creating selfbots [Based on discord.js v13]",
|
||||
"main": "./src/index.js",
|
||||
"types": "./typings/index.d.ts",
|
||||
@ -63,7 +63,6 @@
|
||||
"chalk": "^4.1.2",
|
||||
"discord-api-types": "^0.36.0",
|
||||
"discord-bettermarkdown": "^1.2.0",
|
||||
"discord-rpc-contructor": "^1.1.5",
|
||||
"form-data": "^4.0.0",
|
||||
"json-bigint": "^1.0.0",
|
||||
"node-fetch": "^2.6.1",
|
||||
|
@ -135,6 +135,12 @@ exports.ReactionCollector = require('./structures/ReactionCollector');
|
||||
exports.ReactionEmoji = require('./structures/ReactionEmoji');
|
||||
exports.RichPresenceAssets = require('./structures/Presence').RichPresenceAssets;
|
||||
exports.Role = require('./structures/Role').Role;
|
||||
// RPC
|
||||
exports.getUUID = require('./structures/RichPresence').getUUID;
|
||||
exports.CustomStatus = require('./structures/RichPresence').CustomStatus;
|
||||
exports.RichPresence = require('./structures/RichPresence').RichPresence;
|
||||
exports.SpotifyRPC = require('./structures/RichPresence').SpotifyRPC;
|
||||
//
|
||||
exports.SelectMenuInteraction = require('./structures/SelectMenuInteraction');
|
||||
exports.StageChannel = require('./structures/StageChannel');
|
||||
exports.StageInstance = require('./structures/StageInstance').StageInstance;
|
||||
|
512
src/structures/RichPresence.js
Normal file
512
src/structures/RichPresence.js
Normal file
@ -0,0 +1,512 @@
|
||||
'use strict';
|
||||
const crypto = require('crypto');
|
||||
const { ActivityTypes } = require('../util/Constants');
|
||||
const { resolvePartialEmoji } = require('../util/Util');
|
||||
|
||||
// eslint-disable-next-line
|
||||
const getUUID = () =>
|
||||
([1e7] + -1e3 + -4e3 + -8e3 + -1e11).replace(/[018]/g, a => (a ^ ((Math.random() * 16) >> (a / 4))).toString(16));
|
||||
// Function check url valid (ok copilot)
|
||||
// eslint-disable-next-line
|
||||
const checkUrl = url => /^(?:http(s)?:\/\/)?[\w.-]+(?:\.[\w\.-]+)+[\w\-\._~:/?#[\]@!\$&'\(\)\*\+,;=.]+$/.test(url);
|
||||
|
||||
class CustomStatus {
|
||||
/**
|
||||
* @typedef {Object} CustomStatusOptions
|
||||
* @property {string} [state] The state to be displayed
|
||||
* @property {EmojiIdentifierResolvable} [emoji] The emoji to be displayed
|
||||
*/
|
||||
|
||||
/**
|
||||
* @param {CustomStatus|CustomStatusOptions} [data={}] CustomStatus to clone or raw data
|
||||
*/
|
||||
constructor(data = {}) {
|
||||
this.name = 'Custom Status';
|
||||
/**
|
||||
* The emoji to be displayed
|
||||
* @type {?EmojiIdentifierResolvable}
|
||||
*/
|
||||
this.emoji = null;
|
||||
this.type = ActivityTypes.CUSTOM;
|
||||
/**
|
||||
* The state to be displayed
|
||||
* @type {?string}
|
||||
*/
|
||||
this.state = null;
|
||||
this.setup(data);
|
||||
}
|
||||
/**
|
||||
* Sets the status from a JSON object
|
||||
* @param {CustomStatus|CustomStatusOptions} data CustomStatus to clone or raw data
|
||||
* @private
|
||||
*/
|
||||
setup(data) {
|
||||
this.emoji = data.emoji ? resolvePartialEmoji(data.emoji) : null;
|
||||
this.state = data.state;
|
||||
}
|
||||
/**
|
||||
* Set the emoji of this activity
|
||||
* @param {EmojiIdentifierResolvable} emoji The emoji to be displayed
|
||||
* @returns {CustomStatus}
|
||||
*/
|
||||
setEmoji(emoji) {
|
||||
this.emoji = resolvePartialEmoji(emoji);
|
||||
return this;
|
||||
}
|
||||
/**
|
||||
* Set state of this activity
|
||||
* @param {string | null} state The state to be displayed
|
||||
* @returns {CustomStatus}
|
||||
*/
|
||||
setState(state) {
|
||||
this.state = state;
|
||||
return this;
|
||||
}
|
||||
|
||||
/**
|
||||
* Returns an object that can be used to set the status
|
||||
* @returns {CustomStatus}
|
||||
* @example
|
||||
*/
|
||||
toJSON() {
|
||||
if (!this.emoji & !this.state) throw new Error('CustomStatus must have at least one of emoji or state');
|
||||
return {
|
||||
name: this.name,
|
||||
emoji: this.emoji,
|
||||
type: this.type,
|
||||
state: this.state,
|
||||
};
|
||||
}
|
||||
}
|
||||
|
||||
class RichPresence {
|
||||
/**
|
||||
* @param {Client} client Discord client
|
||||
* @param {RichPresence} [data={}] RichPresence to clone or raw data
|
||||
*/
|
||||
constructor(client, data = {}) {
|
||||
Object.defineProperty(this, 'client', { value: client });
|
||||
/**
|
||||
* The activity's name
|
||||
* @type {string}
|
||||
*/
|
||||
this.name = null;
|
||||
/**
|
||||
* The activity status's type
|
||||
* @type {ActivityType}
|
||||
*/
|
||||
this.type = ActivityTypes.PLAYING;
|
||||
/**
|
||||
* If the activity is being streamed, a link to the stream
|
||||
* @type {?string}
|
||||
*/
|
||||
this.url = null;
|
||||
/**
|
||||
* The id of the application associated with this activity
|
||||
* @type {?Snowflake}
|
||||
*/
|
||||
this.application_id = null;
|
||||
/**
|
||||
* State of the activity
|
||||
* @type {?string}
|
||||
*/
|
||||
this.state = null;
|
||||
/**
|
||||
* Details about the activity
|
||||
* @type {?string}
|
||||
*/
|
||||
this.details = null;
|
||||
/**
|
||||
* Party of the activity
|
||||
* @type {?ActivityParty}
|
||||
*/
|
||||
this.party = null;
|
||||
/**
|
||||
* Timestamps for the activity
|
||||
* @type {?ActivityTimestamps}
|
||||
*/
|
||||
this.timestamps = null;
|
||||
/**
|
||||
* Assets for rich presence
|
||||
* @type {?RichPresenceAssets}
|
||||
*/
|
||||
this.assets = null;
|
||||
/**
|
||||
* The labels of the buttons of this rich presence
|
||||
* @type {string[]}
|
||||
*/
|
||||
this.buttons = null;
|
||||
this.setup(data);
|
||||
}
|
||||
/**
|
||||
* Sets the status from a JSON object
|
||||
* @param {RichPresence} data data
|
||||
* @private
|
||||
*/
|
||||
setup(data) {
|
||||
this.name = data.name;
|
||||
this.type = typeof data.type != 'number' ? ActivityTypes[data.type?.toUpperCase()] : data.type;
|
||||
this.application_id = data.application_id;
|
||||
this.url = data.url;
|
||||
this.state = data.state;
|
||||
this.details = data.details;
|
||||
this.party = data.party;
|
||||
this.timestamps = data.timestamps;
|
||||
this.created_at = data.created_at;
|
||||
this.secrets = data.secrets;
|
||||
this.assets = data.assets;
|
||||
this.buttons = data.buttons;
|
||||
this.metadata = data.metadata;
|
||||
}
|
||||
/**
|
||||
* Set the large image of this activity
|
||||
* @param {?Snowflake} image The large image asset's id
|
||||
* @returns {RichPresence}
|
||||
*/
|
||||
setAssetsLargeImage(image) {
|
||||
// Gif support
|
||||
if (
|
||||
typeof image == 'string' &&
|
||||
(image.startsWith('https://') || image.startsWith('http://')) &&
|
||||
image.includes('external') &&
|
||||
image.includes('discord')
|
||||
) {
|
||||
image = `mp:external${image.split('external')[1]}`;
|
||||
}
|
||||
if (!(this.assets instanceof Object)) this.assets = {};
|
||||
this.assets.large_image = image;
|
||||
return this;
|
||||
}
|
||||
/**
|
||||
* Set the small image of this activity
|
||||
* @param {?Snowflake} image The small image asset's id
|
||||
* @returns {RichPresence}
|
||||
*/
|
||||
setAssetsSmallImage(image) {
|
||||
// Gif support
|
||||
if (
|
||||
typeof image == 'string' &&
|
||||
(image.startsWith('https://') || image.startsWith('http://')) &&
|
||||
image.includes('external') &&
|
||||
image.includes('discord')
|
||||
) {
|
||||
image = `mp:external${image.split('external')[1]}`;
|
||||
}
|
||||
if (!(this.assets instanceof Object)) this.assets = {};
|
||||
this.assets.small_image = image;
|
||||
return this;
|
||||
}
|
||||
/**
|
||||
* Hover text for the large image
|
||||
* @param {string} text Assets text
|
||||
* @returns {RichPresence}
|
||||
*/
|
||||
setAssetsLargeText(text) {
|
||||
if (typeof this.assets !== 'object') this.assets = {};
|
||||
this.assets.large_text = text;
|
||||
return this;
|
||||
}
|
||||
/**
|
||||
* Hover text for the small image
|
||||
* @param {string} text Assets text
|
||||
* @returns {RichPresence}
|
||||
*/
|
||||
setAssetsSmallText(text) {
|
||||
if (typeof this.assets !== 'object') this.assets = {};
|
||||
this.assets.small_text = text;
|
||||
return this;
|
||||
}
|
||||
/**
|
||||
* Set the name of the activity
|
||||
* @param {?string} name The activity's name
|
||||
* @returns {RichPresence}
|
||||
*/
|
||||
setName(name) {
|
||||
this.name = name;
|
||||
return this;
|
||||
}
|
||||
/**
|
||||
* If the activity is being streamed, a link to the stream
|
||||
* @param {?string} url URL of the stream
|
||||
* @returns {RichPresence}
|
||||
*/
|
||||
setURL(url) {
|
||||
if (typeof url == 'string' && !checkUrl(url)) throw new Error('URL must be a valid URL');
|
||||
if (typeof url != 'string') url = null;
|
||||
this.url = url;
|
||||
return this;
|
||||
}
|
||||
/**
|
||||
* The activity status's type
|
||||
* @param {?ActivityTypes} type The type of activity
|
||||
* @returns {RichPresence}
|
||||
*/
|
||||
setType(type) {
|
||||
this.type = ActivityTypes[type?.toUpperCase()];
|
||||
if (typeof this.type == 'string') this.type = ActivityTypes[this.type];
|
||||
if (typeof this.type != 'number') throw new Error('Type must be a valid ActivityType');
|
||||
return this;
|
||||
}
|
||||
/**
|
||||
* Set the application id of this activity
|
||||
* @param {?Snowflake} id Bot's id
|
||||
* @returns {RichPresence}
|
||||
*/
|
||||
setApplicationId(id) {
|
||||
this.application_id = id;
|
||||
return this;
|
||||
}
|
||||
/**
|
||||
* Set the state of the activity
|
||||
* @param {?string} state The state of the activity
|
||||
* @returns {RichPresence}
|
||||
*/
|
||||
setState(state) {
|
||||
this.state = state;
|
||||
return this;
|
||||
}
|
||||
/**
|
||||
* Set the details of the activity
|
||||
* @param {?string} details The details of the activity
|
||||
* @returns {RichPresence}
|
||||
*/
|
||||
setDetails(details) {
|
||||
this.details = details;
|
||||
return this;
|
||||
}
|
||||
/**
|
||||
* @typedef {Object} RichParty
|
||||
* @property {string} id The id of the party
|
||||
* @property {number} max The maximum number of members in the party
|
||||
* @property {number} current The current number of members in the party
|
||||
*/
|
||||
/**
|
||||
* Set the party of this activity
|
||||
* @param {?RichParty} party The party to be displayed
|
||||
* @returns {RichPresence}
|
||||
*/
|
||||
setParty(party) {
|
||||
if (typeof party == 'object') {
|
||||
if (!party.max || typeof party.max != 'number') throw new Error('Party must have max number');
|
||||
if (!party.current || typeof party.current != 'number') throw new Error('Party must have current');
|
||||
if (party.current > party.max) throw new Error('Party current must be less than max number');
|
||||
if (!party.id || typeof party.id != 'string') party.id = getUUID();
|
||||
this.party = {
|
||||
size: [party.current, party.max],
|
||||
id: party.id,
|
||||
};
|
||||
} else {
|
||||
this.party = null;
|
||||
}
|
||||
return this;
|
||||
}
|
||||
/**
|
||||
* Sets the start timestamp of the activity
|
||||
* @param {?number} timestamp The timestamp of the start of the activity
|
||||
* @returns {RichPresence}
|
||||
*/
|
||||
setStartTimestamp(timestamp) {
|
||||
if (!this.timestamps) this.timestamps = {};
|
||||
this.timestamps.start = timestamp;
|
||||
return this;
|
||||
}
|
||||
/**
|
||||
* Sets the end timestamp of the activity
|
||||
* @param {?number} timestamp The timestamp of the end of the activity
|
||||
* @returns {RichPresence}
|
||||
*/
|
||||
setEndTimestamp(timestamp) {
|
||||
if (!this.timestamps) this.timestamps = {};
|
||||
this.timestamps.end = timestamp;
|
||||
return this;
|
||||
}
|
||||
/**
|
||||
* @typedef {object} RichButton
|
||||
* @property {string} name The name of the button
|
||||
* @property {string} url The url of the button
|
||||
*/
|
||||
/**
|
||||
* Set the buttons of the rich presence
|
||||
* @param {...?RichButton} button A list of buttons to set
|
||||
* @returns {RichPresence}
|
||||
*/
|
||||
setButtons(...button) {
|
||||
if (button.length == 0) {
|
||||
this.buttons = null;
|
||||
delete this.metadata;
|
||||
return this;
|
||||
} else if (button.length > 2) {
|
||||
throw new Error('RichPresence can only have up to 2 buttons');
|
||||
}
|
||||
this.buttons = [];
|
||||
this.metadata = {
|
||||
button_urls: [],
|
||||
};
|
||||
button.flat(2).forEach(b => {
|
||||
if (b.name && b.url) {
|
||||
this.buttons.push(b.name);
|
||||
if (!checkUrl(b.url)) throw new Error('Button url must be a valid url');
|
||||
this.metadata.button_urls.push(b.url);
|
||||
} else {
|
||||
throw new Error('Button must have name and url');
|
||||
}
|
||||
});
|
||||
return this;
|
||||
}
|
||||
/**
|
||||
* Add a button to the rich presence
|
||||
* @param {string} name The name of the button
|
||||
* @param {string} url The url of the button
|
||||
* @returns {RichPresence}
|
||||
*/
|
||||
addButton(name, url) {
|
||||
if (!name || !url) {
|
||||
throw new Error('Button must have name and url');
|
||||
}
|
||||
if (typeof name !== 'string') throw new Error('Button name must be a string');
|
||||
if (!checkUrl(url)) throw new Error('Button url must be a valid url');
|
||||
if (!this.buttons) {
|
||||
this.buttons = [];
|
||||
this.metadata = {
|
||||
button_urls: [],
|
||||
};
|
||||
}
|
||||
this.buttons.push(name);
|
||||
this.metadata.button_urls.push(url);
|
||||
return this;
|
||||
}
|
||||
/**
|
||||
* Convert the rich presence to a JSON object
|
||||
* @returns {RichPresence}
|
||||
*/
|
||||
toJSON() {
|
||||
return {
|
||||
name: this.name,
|
||||
type: this.type,
|
||||
application_id: this.application_id,
|
||||
url: this.url,
|
||||
state: this.state,
|
||||
details: this.details,
|
||||
party: this.party,
|
||||
timestamps: this.timestamps,
|
||||
secrets: this.secrets,
|
||||
assets: this.assets,
|
||||
buttons: this.buttons,
|
||||
metadata: this.metadata,
|
||||
};
|
||||
}
|
||||
}
|
||||
|
||||
class SpotifyRPC extends RichPresence {
|
||||
/**
|
||||
* Create a new RichPresence (Spotify style)
|
||||
* @param {Client} client Discord Client
|
||||
* @param {SpotifyRPC} options Options for the Spotify RPC
|
||||
*/
|
||||
constructor(client, options = {}) {
|
||||
if (!client) throw new Error('Client must be set');
|
||||
super(client, options);
|
||||
this.setup(options);
|
||||
}
|
||||
/**
|
||||
* Sets the status from a JSON object
|
||||
* @param {SpotifyRPC} options data
|
||||
* @private
|
||||
*/
|
||||
setup(options) {
|
||||
this.party = {
|
||||
id: `spotify:${this.client.user.id}`,
|
||||
};
|
||||
/**
|
||||
* The Spotify song's id
|
||||
* @type {?string}
|
||||
*/
|
||||
this.sync_id = options.sync_id;
|
||||
/**
|
||||
* The activity's id
|
||||
* @type {string}
|
||||
*/
|
||||
this.id = 'spotify:1';
|
||||
/**
|
||||
* Creation date of the activity
|
||||
* @type {number}
|
||||
*/
|
||||
this.created_at = Date.now();
|
||||
/**
|
||||
* Flags that describe the activity
|
||||
* @type {Readonly<ActivityFlags>}
|
||||
*/
|
||||
this.flags = 48; // Sync + Play (ActivityFlags)
|
||||
/**
|
||||
* The game's or Spotify session's id
|
||||
* @type {?string}
|
||||
*/
|
||||
this.session_id = this.client.ws.shards.first().sessionId;
|
||||
|
||||
this.secrets = {
|
||||
join: crypto.randomBytes(20).toString('hex'), // SHA1 / SHA128
|
||||
spectate: crypto.randomBytes(20).toString('hex'),
|
||||
match: crypto.randomBytes(20).toString('hex'),
|
||||
};
|
||||
}
|
||||
setAssetsLargeImage(image) {
|
||||
if (image.startsWith('spotify:')) image = image.replace('spotify:', '');
|
||||
if (!(this.assets instanceof Object)) {
|
||||
this.assets = {
|
||||
large_image: `spotify:${image}`,
|
||||
};
|
||||
} else {
|
||||
this.assets.large_image = `spotify:${image}`;
|
||||
}
|
||||
return this;
|
||||
}
|
||||
setAssetsSmallImage(image) {
|
||||
if (image.startsWith('spotify:')) image = image.replace('spotify:', '');
|
||||
if (!(this.assets instanceof Object)) {
|
||||
this.assets = {
|
||||
small_image: `spotify:${image}`,
|
||||
};
|
||||
} else {
|
||||
this.assets.small_image = `spotify:${image}`;
|
||||
}
|
||||
return this;
|
||||
}
|
||||
/**
|
||||
* Set Spotify song id to sync with
|
||||
* @param {string} id Song id
|
||||
* @returns {SpotifyRPC}
|
||||
*/
|
||||
setSongId(id) {
|
||||
this.sync_id = id;
|
||||
return this;
|
||||
}
|
||||
|
||||
toJSON() {
|
||||
if (!this.sync_id) throw new Error('Song id is required');
|
||||
return {
|
||||
name: 'Spotify',
|
||||
type: ActivityTypes.LISTENING,
|
||||
application_id: this.application_id,
|
||||
url: this.url,
|
||||
state: this.state,
|
||||
details: this.details,
|
||||
party: this.party,
|
||||
timestamps: this.timestamps,
|
||||
secrets: this.secrets,
|
||||
assets: this.assets,
|
||||
session_id: this.session_id,
|
||||
sync_id: this.sync_id,
|
||||
flags: this.flags,
|
||||
id: this.id,
|
||||
created_at: this.created_at,
|
||||
metadata: this.metadata,
|
||||
};
|
||||
}
|
||||
}
|
||||
|
||||
module.exports = {
|
||||
CustomStatus,
|
||||
RichPresence,
|
||||
SpotifyRPC,
|
||||
getUUID,
|
||||
};
|
85
typings/index.d.ts
vendored
85
typings/index.d.ts
vendored
@ -165,6 +165,91 @@ import DiscordAuthWebsocket from '../src/util/RemoteAuth.js';
|
||||
|
||||
//#region Classes
|
||||
|
||||
// RPC by aiko-chan-ai
|
||||
export interface RichButton {
|
||||
name: string;
|
||||
url: string;
|
||||
}
|
||||
|
||||
export abstract class RichPresence {
|
||||
public constructor(client?: Client, data?: object);
|
||||
public application_id: Snowflake | null;
|
||||
public assets: RichPresenceAssets | null;
|
||||
public buttons: string[];
|
||||
public details: string | null;
|
||||
public name: string;
|
||||
public party: {
|
||||
id: string | null;
|
||||
size: [number, number];
|
||||
} | null;
|
||||
public state: string | null;
|
||||
public timestamps: {
|
||||
start: Date | null;
|
||||
end: Date | null;
|
||||
} | null;
|
||||
public type: ActivityType;
|
||||
public url: string | null;
|
||||
public setAssetsLargeImage(image?: Snowflake): this;
|
||||
public setAssetsLargeText(text?: string): this;
|
||||
public setAssetsSmallImage(image?: Snowflake): this;
|
||||
public setAssetsSmallText(text?: string): this;
|
||||
public setName(name?: string): this;
|
||||
public setURL(url?: string): this;
|
||||
public setType(type?: ActivityType): this;
|
||||
public setApplicationId(id?: Snowflake): this;
|
||||
public setDetails(details?: string): this;
|
||||
public setState(state?: string): this;
|
||||
public setParty(party?: { max: number; current: number; id?: string }): this;
|
||||
public setStartTimestamp(timestamp?: Date): this;
|
||||
public setEndTimestamp(timestamp?: Date): this;
|
||||
public setButtons(...button: RichButton[]): this;
|
||||
public addButton(name: string, url: string): this;
|
||||
public toJSON(): object;
|
||||
}
|
||||
|
||||
export abstract class SpotifyRPC extends RichPresence {
|
||||
public constructor(client: Client, data?: object);
|
||||
public application_id: Snowflake | null;
|
||||
public client: Client;
|
||||
public assets: RichPresenceAssets | null;
|
||||
public buttons: string[];
|
||||
public details: string | null;
|
||||
public name: string;
|
||||
public sync_id: string;
|
||||
public id: string;
|
||||
public created_at: Date;
|
||||
public flags: number;
|
||||
public secrets: {
|
||||
join: string;
|
||||
spectate: string;
|
||||
match: string;
|
||||
};
|
||||
public session_id: string;
|
||||
public party: {
|
||||
id: string | null;
|
||||
size: [number, number];
|
||||
} | null;
|
||||
public state: string | null;
|
||||
public timestamps: {
|
||||
start: Date | null;
|
||||
end: Date | null;
|
||||
} | null;
|
||||
public type: ActivityType;
|
||||
public url: string | null;
|
||||
public setAssetsLargeImage(image?: string): this;
|
||||
public setAssetsSmallImage(image?: string): this;
|
||||
public setSongId(id: string): this;
|
||||
}
|
||||
|
||||
export abstract class CustomStatus {
|
||||
public constructor(data?: object);
|
||||
public emoji: EmojiIdentifierResolvable;
|
||||
public state: string;
|
||||
public setEmoji(emoji?: EmojiIdentifierResolvable): this;
|
||||
public setState(state: string): this;
|
||||
public toJSON(): object;
|
||||
}
|
||||
|
||||
export class Activity {
|
||||
private constructor(presence: Presence, data?: RawActivityData);
|
||||
public applicationId: Snowflake | null;
|
||||
|
Loading…
x
Reference in New Issue
Block a user